Is it vibe coding if I know what I’m doing?
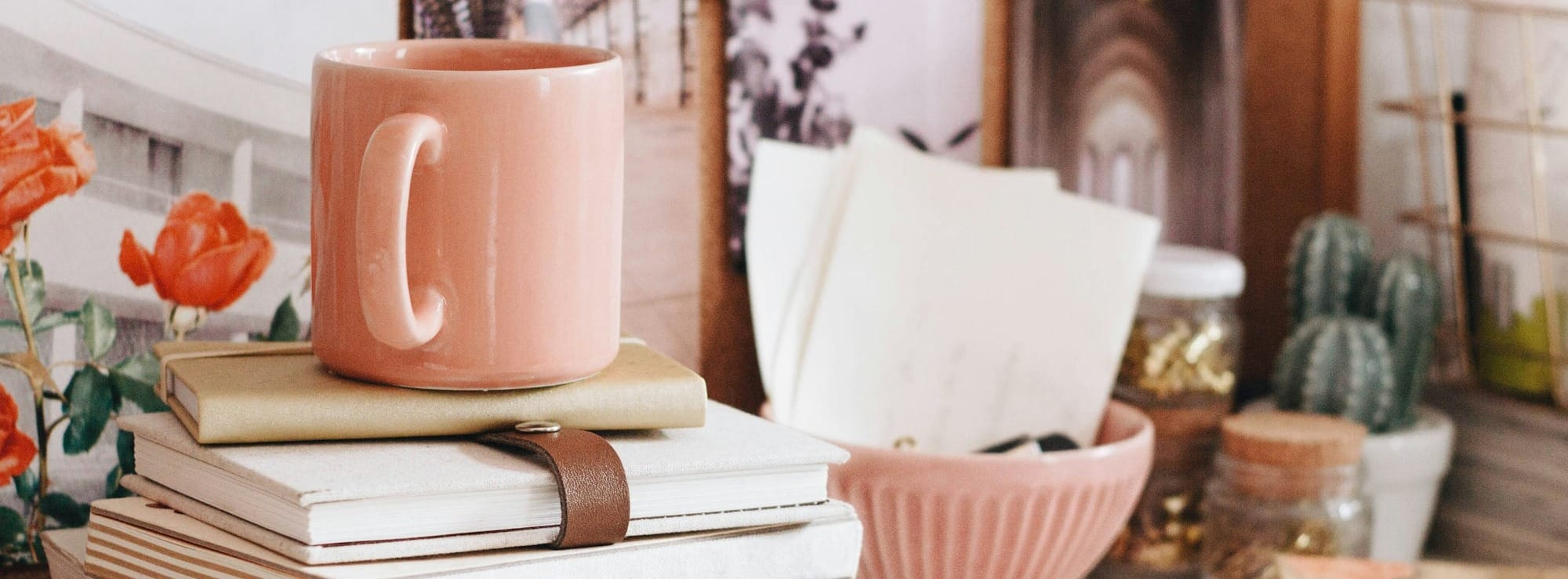
Anyone can watch a YouTube video and think they can renovate their bathroom, but as someone who has tried to do it, I can tell you that it’s a lot harder than it looks. I think vibe coding is the same way. Anyone can ask Lovable or Bolt to try and create an application, but if you don’t know what you’re doing, how do you know that it’s right?
As a professional programmer, is it vibe coding if I know what I'm doing?
As an experiment, I thought I'd try creating an app using Chatgpt and see how much time it would save me. But where to start?
Brew seemed to be struggling on my MacBook Pro. So I started by asking Chatgpt:
I needed to clean up script for Brew.
It produced the script, which I’ve seen before as a gist.
# Update Homebrew itself
echo "Updating Homebrew..."
$HOMEBREW_PREFIX/bin/brew update
# Upgrade outdated packages
echo "Upgrading outdated packages..."
$HOMEBREW_PREFIX/bin/brew upgrade
# Clean up unused packages
echo "Cleaning up unused packages..."
$HOMEBREW_PREFIX/bin/brew cleanup
# Optional: Upgrade casks (applications) as well
echo "Upgrading outdated casks (applications)..."
$HOMEBREW_PREFIX/bin/brew upgrade --cask
echo "Brew health check complete!"
So I knew how this would work, but I wanted it to be a little bit better, so I asked:
Update the script to be a function and run it only if the
script has not been run in 7 days.
And this is what he gave me, which is what I would expect: dropping a file with the time it last ran and not running it if it’s less than seven days.
#!/bin/bash
# Path to store the timestamp of last run
LAST_RUN_FILE="$HOME/.last_script_run"
# Current time in seconds since epoch
NOW=$(date +%s)
# How many seconds in 7 days
SEVEN_DAYS=$((7 * 24 * 60 * 60))
# Function to update and clean Homebrew
run_brew_maintenance() {
echo "Updating Homebrew..."
$HOMEBREW_PREFIX/bin/brew update
echo "Upgrading outdated packages..."
$HOMEBREW_PREFIX/bin/brew upgrade
echo "Cleaning up unused packages..."
$HOMEBREW_PREFIX/bin/brew cleanup
echo "Upgrading outdated casks (applications)..."
$HOMEBREW_PREFIX/bin/brew upgrade --cask
echo "Brew health check complete!"
}
# Check if the last run file exists
if [ -f "$LAST_RUN_FILE" ]; then
LAST_RUN=$(cat "$LAST_RUN_FILE")
TIME_DIFF=$((NOW - LAST_RUN))
if [ "$TIME_DIFF" -ge "$SEVEN_DAYS" ]; then
echo "The script has NOT been run in the last 7 days. Running maintenance..."
run_brew_maintenance
echo "$NOW" > "$LAST_RUN_FILE"
else
echo "The script was run within the last 7 days. Skipping maintenance."
fi
else
echo "The script has NEVER been run before. Running maintenance..."
run_brew_maintenance
echo "$NOW" > "$LAST_RUN_FILE"
fi
What I liked about this is that I didn’t have to deal with the date math to ensure it was less than seven days. This is all good code, but it would’ve taken me probably 30 minutes to do this myself.
So it runs, but what about running at any time, so I asked:
Add a flag to this script to force it to be run
This is precisely what I would’ve done if I were doing it myself. Chatgpt did it for me in a few seconds, but because I don’t do this every day, it would have taken me another half hour.
#!/bin/bash
# Set this if not defined in your shell environment
HOMEBREW_PREFIX="${HOMEBREW_PREFIX:-/opt/homebrew}"
# Path to store the timestamp of last run
LAST_RUN_FILE="$HOME/.last_script_run"
# Current time in seconds since epoch
NOW=$(date +%s)
# How many seconds in 7 days
SEVEN_DAYS=$((7 * 24 * 60 * 60))
# Parse arguments
FORCE_RUN=false
if [[ "$1" == "--force" ]]; then
FORCE_RUN=true
fi
# Function to update and clean Homebrew
run_brew_maintenance() {
echo "Updating Homebrew..."
$HOMEBREW_PREFIX/bin/brew update
echo "Upgrading outdated packages..."
$HOMEBREW_PREFIX/bin/brew upgrade
echo "Cleaning up unused packages..."
$HOMEBREW_PREFIX/bin/brew cleanup
echo "Upgrading outdated casks (applications)..."
$HOMEBREW_PREFIX/bin/brew upgrade --cask
echo "Brew health check complete!"
}
# Main logic
if $FORCE_RUN; then
echo "Forced run triggered. Running maintenance..."
run_brew_maintenance
echo "$NOW" > "$LAST_RUN_FILE"
else
if [ -f "$LAST_RUN_FILE" ]; then
LAST_RUN=$(cat "$LAST_RUN_FILE")
TIME_DIFF=$((NOW - LAST_RUN))
if [ "$TIME_DIFF" -ge "$SEVEN_DAYS" ]; then
echo "The script has NOT been run in the last 7 days. Running maintenance..."
run_brew_maintenance
echo "$NOW" > "$LAST_RUN_FILE"
else
echo "The script was run within the last 7 days. Skipping maintenance."
fi
else
echo "The script has NEVER been run before. Running maintenance..."
run_brew_maintenance
echo "$NOW" > "$LAST_RUN_FILE"
fi
fi
I don’t want to allow this script to run if it's currently running.
only run the script if it is not currently running
And of course, it created a lock file, and if lock file is there it won’t run.
#!/bin/bash
# Set this if not defined in your shell environment
HOMEBREW_PREFIX="${HOMEBREW_PREFIX:-/opt/homebrew}"
# Lock file to prevent concurrent runs
LOCKFILE="/tmp/$(basename "$0").lock"
# Timestamp storage file
LAST_RUN_FILE="$HOME/.last_script_run"
# Current time in seconds since epoch
NOW=$(date +%s)
# How many seconds in 7 days
SEVEN_DAYS=$((7 * 24 * 60 * 60))
# Parse arguments
FORCE_RUN=false
if [[ "$1" == "--force" ]]; then
FORCE_RUN=true
fi
# Function to update and clean Homebrew
run_brew_maintenance() {
echo "Updating Homebrew..."
$HOMEBREW_PREFIX/bin/brew update
echo "Upgrading outdated packages..."
$HOMEBREW_PREFIX/bin/brew upgrade
echo "Cleaning up unused packages..."
$HOMEBREW_PREFIX/bin/brew cleanup
echo "Upgrading outdated casks (applications)..."
$HOMEBREW_PREFIX/bin/brew upgrade --cask
echo "Brew health check complete!"
}
# Acquire lock
if [ -e "$LOCKFILE" ]; then
echo "Script is already running. Exiting."
exit 1
else
# Create lock file and remove on exit
touch "$LOCKFILE"
trap 'rm -f "$LOCKFILE"' EXIT
fi
# Main logic
if $FORCE_RUN; then
echo "Forced run triggered. Running maintenance..."
run_brew_maintenance
echo "$NOW" > "$LAST_RUN_FILE"
else
if [ -f "$LAST_RUN_FILE" ]; then
LAST_RUN=$(cat "$LAST_RUN_FILE")
TIME_DIFF=$((NOW - LAST_RUN))
if [ "$TIME_DIFF" -ge "$SEVEN_DAYS" ]; then
echo "The script has NOT been run in the last 7 days. Running maintenance..."
run_brew_maintenance
echo "$NOW" > "$LAST_RUN_FILE"
else
echo "The script was run within the last 7 days. Skipping maintenance."
fi
else
echo "The script has NEVER been run before. Running maintenance..."
run_brew_maintenance
echo "$NOW" > "$LAST_RUN_FILE"
fi
fi
This saved me about 15 minutes.
But what happens if the script crashes and leaves the lock file behind:
Add a cleanup flag to remove the lock file and run the script
Since I already looked up how to create a flag that probably would’ve taken me another five minutes.
#!/bin/bash
# Set this if not defined in your shell environment
HOMEBREW_PREFIX="${HOMEBREW_PREFIX:-/opt/homebrew}"
# Lock file to prevent concurrent runs
LOCKFILE="/tmp/$(basename "$0").lock"
# Timestamp storage file
LAST_RUN_FILE="$HOME/.last_script_run"
# Current time in seconds since epoch
NOW=$(date +%s)
# How many seconds in 7 days
SEVEN_DAYS=$((7 * 24 * 60 * 60))
# Parse arguments
FORCE_RUN=false
CLEANUP_LOCK=false
while [[ "$1" != "" ]]; do
case $1 in
--force ) FORCE_RUN=true ;;
--cleanup ) CLEANUP_LOCK=true ;;
esac
shift
done
# If cleanup is requested, remove the lock file before proceeding
if $CLEANUP_LOCK; then
echo "Cleaning up stale lock file (if exists)..."
rm -f "$LOCKFILE"
fi
# Function to update and clean Homebrew
run_brew_maintenance() {
echo "Updating Homebrew..."
$HOMEBREW_PREFIX/bin/brew update
echo "Upgrading outdated packages..."
$HOMEBREW_PREFIX/bin/brew upgrade
echo "Cleaning up unused packages..."
$HOMEBREW_PREFIX/bin/brew cleanup
echo "Upgrading outdated casks (applications)..."
$HOMEBREW_PREFIX/bin/brew upgrade --cask
echo "Brew health check complete!"
}
# Acquire lock
if [ -e "$LOCKFILE" ]; then
echo "Script is already running. Exiting."
exit 1
else
# Create lock file and ensure it's removed on exit
touch "$LOCKFILE"
trap 'rm -f "$LOCKFILE"' EXIT
fi
# Main logic
if $FORCE_RUN; then
echo "Forced run triggered. Running maintenance..."
run_brew_maintenance
echo "$NOW" > "$LAST_RUN_FILE"
else
if [ -f "$LAST_RUN_FILE" ]; then
LAST_RUN=$(cat "$LAST_RUN_FILE")
TIME_DIFF=$((NOW - LAST_RUN))
if [ "$TIME_DIFF" -ge "$SEVEN_DAYS" ]; then
echo "The script has NOT been run in the last 7 days. Running maintenance..."
run_brew_maintenance
echo "$NOW" > "$LAST_RUN_FILE"
else
echo "The script was run within the last 7 days. Skipping maintenance."
fi
else
echo "The script has NEVER been run before. Running maintenance..."
run_brew_maintenance
echo "$NOW" > "$LAST_RUN_FILE"
fi
fi
So now I have my script ready to go, and it also created some instructions for me on how to use it and added it to the top of the script.
Created instructions on how to use this script and add it to the beginning
Which I never do.
# Usage: ./brew_health.sh [options]
# Options:
# --force Run maintenance regardless of last run time
# --cleanup Remove any stale lock file before running
# --dry-run Show what would be done without making changes
# --status Show when the script was last run
#
# This script performs Homebrew maintenance tasks including:
# - Updating Homebrew
# - Upgrading outdated packages
# - Cleaning up unused packages
# - Upgrading outdated casks (applications)
#
# The script will only run if it hasn't been run in the last 7 days,
# unless the --force option is used.
Now I have a pretty fabulous brew cleanup script
add some colors to the output
And now I have this:
which I've put on gist
So, this is the application I wanted, but am I a vibe coder? I mean, each step produces the exact code that I would have created if I did it myself, but it would have taken me an hour, and this took me about 10 minutes.
So, my outcome from this is that Chatgpt and other LLMS can create code much faster than I can, but I still need to evaluate it to make sure it’s right. I’m not gonna trust anything right, it’s gotta look right or else I’m not gonna use it.
So I pretty much feel like my job is safe, just like with my bathroom reno. Things aren’t gonna work right, and eventually, you’re gonna have to go and call a professional to fix it.